Flutter: диалоговое окно
В предыдущей статье, мы нарисовали меню. Теперь нужно начинать реализовывать какую-то реакцию на действия в этом меню. Например показать диалоговое окно закрытия приложения. Это правило хорошего тона у приложений — спросить прежде чем окончательно выйти.
Во Flutter за показ диалогов отвечают две фунции, которые работают непосредственно друг с другом в связке:
AlertDialog — подготовка данных для отображения
showDialog — непосредственные вывод диалога пользователю
Оформим диалог в виде отдельно функции:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
SureExitDialog(BuildContext context) { Widget cancelButton = TextButton( child: Text("Остаться"), onPressed: () {Navigator.pop(context);}, ); Widget yesButton = TextButton( child: Text("Да"), onPressed: () { if (Platform.isAndroid) { SystemChannels.platform.invokeMethod( 'SystemNavigator.pop'); } else { exit(0); }; }, ); AlertDialog alert = AlertDialog( title: Text("Подтверждение"), content: Text("Вы действительно хотите выйти из приложения?"), actions: [ cancelButton, yesButton, ], ); showDialog( context: context, builder: (BuildContext context) { return alert; }, ); } |
Добавим вызов диалога из нажатия кнопки выхода:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
@override Widget build(BuildContext context) { return Scaffold( key: scaffoldKey, appBar: AppBar( backgroundColor: Colors.green, automaticallyImplyLeading: true, title: Text( 'Инвентаризация' ), actions: [ new IconButton( onPressed: () { SureExitDialog(context); }, icon: new Icon(Icons.exit_to_app) ), ], centerTitle: true, elevation: 4, ), ... |
Результат:
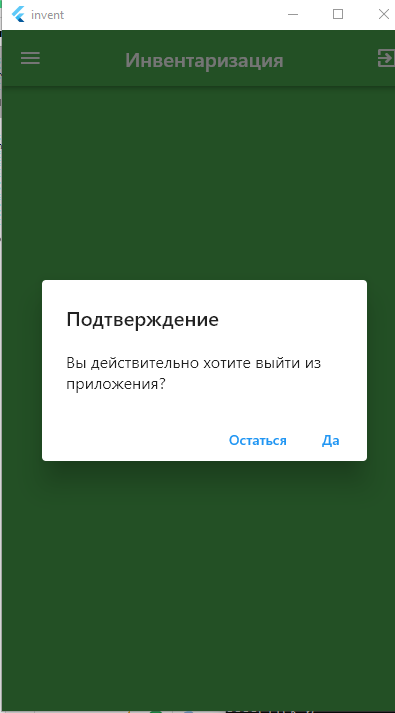
Для любителей диалоговых окон во весь экран (что актуально для мобильных, вот еще вариант:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
showDialog( context: context, builder: (BuildContext context) { return Align ( alignment: Alignment.bottomCenter, child: Container( width: double.infinity, decoration: BoxDecoration( color: Colors.white, borderRadius: BorderRadius.only( topLeft: Radius.circular(32.0), topRight: Radius.circular(32.0))), child:Column( mainAxisSize: MainAxisSize.min, mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Padding( padding: const EdgeInsets.all(16.0), child: Material( child: Text("Подтверждение", style: TextStyle( fontSize: 14.0, color: Colors.black))), ), Padding( padding: const EdgeInsets.all(16.0), child: Material( child: Text("Вы действительно хотите выйти из приложения?", style: TextStyle( fontSize: 14.0, color: Colors.black))), ), cancelButton, yesButton ] ) ) ); }, ); } |
Результат:
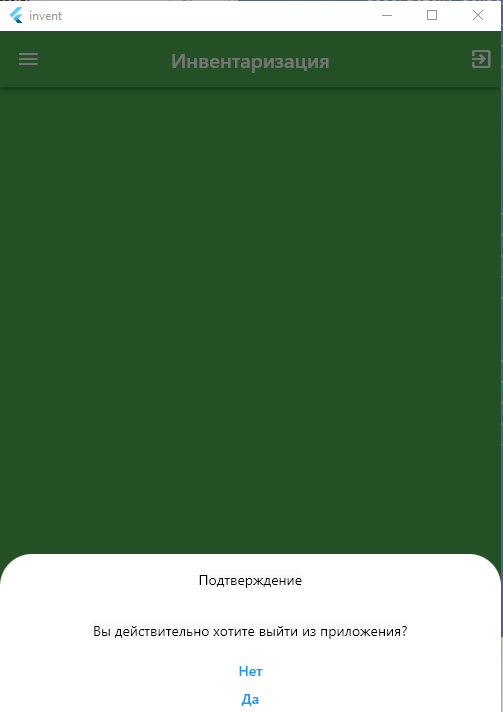